I didn’t set out to build WebKit with VS2012, but that’s exactly what I ended up doing anyway…
I just built a new workstation from scratch. Clean as a baby’s memory, I thought it’d be fun to taste my own dog food. Starting with a newly installed Windows 7 64-bit and my previous walk-through I set to build WebKit.
Software Setup
- First, I downloaded the Cygwin downloader from here and ran it. Once complete, I ran setup.exe, gave it a target path and selected to install all packages.
- Next, I installed Visual Studio 2010. We really care about C++ tool chain. But other features may be safely installed.
- Windows SDK 7.1 was installed next. I chose to install the x64 version, of course. Again, we need only C++ for x64, but installing other stuff is fine.
- Now I could install Visual Studio 2010 SP1.
- And finally the fix to restore Visual C++ 2010 SP1 Compiler Update for the Windows SDK 7.1 (because the SDK installs pre-SP1 files).
Notes:
Cygwin has warned that the latest version (1.7) is a major update over the previous one. This sounded more like a hint that things might break. As we’ll see, this was not a vain warning.
The order for installing Visual Studio and updates is important.
Preparation
I decided to attempt first to build what I knew to be a good source (with a large project like this, things break fast,) so i started with r106194. After extracting the archive, I copied the environment setup script (aptly called vs2010-build-env.cmd) from the previous post. Reviewed the Cygwin source path to be valid (I had to update it because I’ve since replaced Tools folder with Bin). Running the vs2010-build-env.cmd script As Administrator, I got a bash shell.
First attempt failed with a new error:
$ build-webkit --wincairo
Can't locate HTTP/Date.pm in @INC (@INC contains: /usr/lib/perl5/site_perl/5.14/i686-cygwin-threads-64int /usr/lib/perl5/site_perl/5.14 /usr/lib/perl5/vendor_perl/5.14/i686-cygwin-threads-64int /usr/lib/perl5/vendor_perl/5.14 /usr/lib/perl5/5.14/i686-cygwin-threads-64int /usr/lib/pe
rl5/5.14 /usr/lib/perl5/site_perl/5.10 /usr/lib/perl5/vendor_perl/5.10 /usr/lib/perl5/site_perl/5.8 .) at /cygdrive/c/Prj/WebKit/WebKit-r106194/Tools/Scripts/update-webkit-dependency line 39.
BEGIN failed--compilation aborted at /cygdrive/c/Prj/WebKit/WebKit-r106194/Tools/Scripts/update-webkit-dependency line 39.
Died at Tools/Scripts/update-webkit-wincairo-libs line 40.
Died at /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/Scripts/build-webkit line 561.
Cygwin’s Perl package is missing the HTTP/Date.pm module. CPAN to the rescue:
$ cpan HTTP::Date
CPAN.pm requires configuration, but most of it can be done automatically.
If you answer 'no' below, you will enter an interactive dialog for each
configuration option instead.
Would you like to configure as much as possible automatically? [yes]
[Snipped a lot of CPAN output]
Running make install
Installing /usr/lib/perl5/site_perl/5.14/HTTP/Date.pm
Installing /usr/share/man/man3/HTTP.Date.3pm
Appending installation info to /usr/lib/perl5/5.14/i686-cygwin-threads-64int/perllocal.pod
GAAS/HTTP-Date-6.02.tar.gz
/usr/bin/make install -- OK
Let’s try again:
$ build-webkit --wincairo
Checking Last-Modified date of WinCairoRequirements.zip...
Couldn't check Last-Modified date of new WinCairoRequirements.zip.
Please ensure that http://idisk.mac.com/bfulgham-Public/WinCairoRequirements.zip is reachable.
Unable to check Last-Modified date and no version of WinCairoRequirements to fall back to.
Died at Tools/Scripts/update-webkit-wincairo-libs line 40.
Died at /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/Scripts/build-webkit line 561.
Apparently sometime since I built last in April and today Brent Fulgham’s mac.com page went dead. He is the maintainer and host of WinCairoRequirements. I had to either resurrect an old version from my backups or point the script to WinCairoRequirement’s new home. I chose to start with the latter. The updated script shows the new location of the archive to be http://dl.dropbox.com/u/39598926/WinCairoRequirements.zip. But replacing the old URL with the new one wouldn’t work. Apparently DropBox doesn’t report the last-modified date of a file, which is necessary to detect updates, so a workaround is also added in the relevant scripts. Seems that I need to move to newer source revision after all.
Before giving up on the old revision (which I know builds without errors) I decided to do manually what the update script does automatically. I copied the necessary WinCairoRequirement files from an old backup and commented out the perl update-webkit-wincairo-libs script.
# Updates a development environment to the new WebKitAuxiliaryLibrary
use strict;
use warnings;
use FindBin;
my $file = "WinCairoRequirements";
my $zipFile = "$file.zip";
my $winCairoLibsURL = "http://dl.dropbox.com/u/39598926/$zipFile";
my $command = "$FindBin::Bin/update-webkit-dependency";
#system("perl", $command, $winCairoLibsURL, ".") == 0 or die;
Now, I could see the build script complaining that it couldn’t find Visual Studio tools directory. It’s time to update the pdevenv script with support for VS2010. After replacing the pdevenv script with the one provided in my patch zip from the last post I built again. (Well, actually, I cheated: I have a newer and improved version of pdevenv script… more on that later.)
Elevated Mode
It’d be really nice if it were possible to request elevation automatically. There doesn’t seem to be a standard way of doing this, but Johannes Passing has created a tiny, but supremely useful, tool called Elevate
to do just that.
Simply place the binary somewhere on your path (I have a special directory for similar utilities) and call pass the script file path as a parameter to it. There are many ways to use it. One is to create a single-line bat/cmd file with “Elevate cmd
” in it. From the new prompt, one would call vs2010-build-env.cmd. Another solution is to place Elevate
before the mklink
calls in vs2010-build-env.cmd. The first has the advantage that you won’t be prompted more than once, but then again, that might elevate your risks as well.
Note: In the previous posts I suggested forcing Visual Studio to run as Administrator by changing the executable’s properties. However, this isn’t necessary, so long that we invoke it from an elevated shell. Because of (or thanks to, rather) the need for elevation to create symbolic link for Cygwin, there is no need to mess with Visual Studio binaries.
Back to WibKit.
Building
Running build-webkit --wincairo
again throws a new error:
C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Platforms\Win32\Microsoft.Cpp.Win32.Targets(153,5): error MSB6001: Invalid command line switch for "CL.exe". Item has already been added. Key in dictionary: 'tmp' Key being added: 'TMP'
A quick search shows that we have duplicate entries in the environment variable. Sounds like another new Cygwin issue. Let’s see what our environment variables look like:
$ printenv | sort
[Snip]
TEMP=/tmp
temp=C:\Users\Ash\AppData\Local\Temp
TERM=cygwin
TMP=/tmp
tmp=C:\Users\Ash\AppData\Local\Temp
[Snip]
Seems that we do have duplicates after all. Previously Cygwin used to upper-case all environment variables, so duplicates that deferred in case only got eliminated. Cl seems to be doing something very similar, only it does detect the duplication and doesn’t silently overwrite one another. What is odd is that the lower-case versions seem to be inherited from Windows, where they are all-caps. Calling set
in cmd
shows all variable names to be in caps. I’m tempted to think that Cygwin is changing the Windows-inherited variables that conflict with its list to lower-case.
The following Perl snippet does the trick:
# Fix up any env variables that differ only in case.
foreach my $key (sort keys(%ENV)) {
my $upper_key = uc($key);
my $dup = $ENV{$upper_key};
if ($dup && ($dup ne $ENV{$key}))
{
# Dups break CL.exe, so we need to remove either one. Since Cygwin seems to make Windows-inherited
# entries lower-case, and since CL plays better with Windows paths, we remove the upper-case one.
# There seems to be a bug in MSBuild.exe in that stays resident across builds, so environment
# variables changes aren't applied to future builds unless it's killed to force respawning.
print "Found duplicate env-var (rm $upper_key): \t$key = $ENV{$key}\t\t$upper_key = $dup\n";
delete $ENV{$upper_key};
}
}
…well, not quite. Turns out MSBuild.exe, which is a background process that visual studio employs for building, needs to be killed so it would run with our modified environment variables. Otherwise, whatever changes we make to the environment variables, MSBuild.exe will ignore and all CL.exe instances will inherit whatever MSBuild.exe has. This is certainly a bug in the design of MSBuild in that it doesn’t detect and update its environment variables. Instead, it just assumes that whatever it has on the first run will be valid on future runs. Clearly an unwarranted assumption.
Let’s try building again after killing MSBuild.exe and with the new script.
Success, At Last
Here is the complete output, with Quiet log-level, for reference:
$ build-webkit --wincairo
Building results into: /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/WebKitBuild
WEBKITOUTPUTDIR is set to: C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild
WEBKITLIBRARIESDIR is set to: C:\Cygwin\home\Ash\WebKit-r106194\WebKitLibraries\win
/cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/Scripts/pdevenv win\WebKit.vcproj\WebKit.sln /build Release_Cairo_CFLite
Current working directory: /cygdrive/c/Prj/WebKit/WebKit-r106194/Source/WebKit
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/autogen.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/JavaScriptCore/make-generated-sources.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/JavaScriptCore/gyp/generate-derived-sources.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/JavaScriptCore/gyp/generate-dtrace-header.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/JavaScriptCore/gyp/run-if-exists.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/JavaScriptCore/gyp/update-info-plist.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/JavaScriptCore/JavaScriptCore.vcproj/JavaScriptCore/build-generated-files.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/ThirdParty/ANGLE/src/compiler/generate_parser.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/ThirdParty/gtest/xcode/Samples/FrameworkSample/runtests.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/ThirdParty/gtest/xcode/Scripts/runtests.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/make-generated-sources.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/move-js-headers.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/gyp/copy-forwarding-and-icu-headers.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/gyp/copy-inspector-resources.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/gyp/generate-derived-sources.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/gyp/generate-webcore-export-file-generator.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/gyp/run-if-exists.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/gyp/streamline-inspector-source.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/gyp/update-info-plist.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/inspector/compile-front-end.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/WebCore.gyp/mac/adjust_visibility.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/WebCore.gyp/mac/check_objc_rename.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/WebCore.vcproj/build-generated-files.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebCore/WebCore.vcproj/migrate-scripts.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Source/WebKit2/win/build-generated-files.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/CygwinDownloader/make-zip.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/EWSTools/boot.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/EWSTools/start-queue.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/iExploder/iexploder-1.7.2/tools/release_src.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/iExploder/iexploder-1.7.2/tools/update_html_tags_from_sources.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/Scripts/webkit-tools-completion.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/WebKitTestRunner/win/build-generated-files.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/WebKitLibraries/win/tools/scripts/auto-version.sh to Unix format ...
dos2unix: converting file /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/WebKitLibraries/win/tools/scripts/feature-defines.sh to Unix format ...
Found duplicate env-var: temp = C:\Users\Ash\AppData\Local\Temp TEMP = /tmp - Deleting temp.
Found duplicate env-var: tmp = C:\Users\Ash\AppData\Local\Temp TMP = /tmp - Deleting tmp.
Running cmd /c "call C:\Bin\CYGWIN~1\tmp\KSVECI~1.CMD"
print() on closed filehandle $fh at /cygdrive/c/Cygwin/home/Ash/WebKit-r106194/Tools/Scripts/pdevenv line 149.
Using VS2010 toolchain.
Upgrading solution file @ C:\Prj\WebKit\WebKit-r106194\Source\WebKit\win\WebKit.vcproj\WebKit-vs2010.sln. Delete this file to force-upgrade all projects.
Build will commence when done. Please be patient.
Setting environment for using Microsoft Visual Studio 2010 x86 tools.
Microsoft (R) Visual Studio Version 10.0.40219.1.
Copyright (C) Microsoft Corp. All rights reserved.
Information:
This project/solution does not require conversion.
Patching common.props...
Disabling warning as error.
Disabling warning C4396.
Enabling multiprocess compile.
Patching JavaScriptCore.def...
1 file(s) moved.
Correcting WebCore.vcxproj...
Setting environment for using Microsoft Visual Studio 2010 x86 tools.
Microsoft (R) Visual Studio Version 10.0.40219.1.
Copyright (C) Microsoft Corp. All rights reserved.
1>------ Build started: Project: JavaScriptCoreGenerated, Configuration: Release_Cairo_CFLite Win32 ------
2>------ Build started: Project: WTF, Configuration: Release_Cairo_CFLite Win32 ------
2>StringExtras.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
2>SizeLimits.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
2>NullPtr.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
2>HashTable.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
2>DynamicAnnotations.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
2>BinarySemaphore.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
3>------ Build started: Project: JavaScriptCore, Configuration: Release_Cairo_CFLite Win32 ------
3>JSGlobalObject.obj : warning LNK4197: export '?s_globalObjectMethodTable@JSGlobalObject@JSC@@1UGlobalObjectMethodTable@2@B' specified multiple times; using first specification
3>PropertyDescriptor.obj : warning LNK4197: export '?defaultAttributes@PropertyDescriptor@JSC@@0IA' specified multiple times; using first specification
3>MachineStackMarker.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>Heap.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSValueRef.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSWeakObjectMapRefPrivate.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>BytecodeGenerator.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>Parser.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSCallbackObject.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSClassRef.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSContextRef.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSObjectRef.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSGlobalData.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSBase.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSCallbackConstructor.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
3>JSCallbackFunction.obj : warning LNK4049: locally defined symbol ?staticData@WTFThreadData@WTF@@0PAV?$ThreadSpecific@VWTFThreadData@WTF@@@2@A (private: static class WTF::ThreadSpecific * WTF::WTFThreadData::staticData) imported
4>------ Build started: Project: WebCoreGenerated, Configuration: Release_Cairo_CFLite Win32 ------
4>C:\Prj\WebKit\WebKit-r106194\Source\WebCore\WebCore.vcproj\WebCoreGeneratedCairo.props(4,5): warning MSB4011: "C:\Prj\WebKit\WebKit-r106194\WebKitLibraries\win\tools\vsprops\common.props" cannot be imported again. It was already imported at "C:\Prj\WebKit\WebKit-r106194\Source\WebCore\WebCore.vcproj\WebCoreGeneratedCommon.props (4,5)". This is most likely a build authoring error. This subsequent import will be ignored. [C:\Prj\WebKit\WebKit-r106194\Source\WebCore\WebCore.vcproj\WebCoreGenerated.vcxproj]
5>------ Skipped Build: Project: QTMovieWin, Configuration: Release_Cairo_CFLite Win32 ------
5>Project not selected to build for this solution configuration
6>------ Build started: Project: WebCore, Configuration: Release_Cairo_CFLite Win32 ------
6>..\platform\graphics\cairo\DrawErrorUnderline.h(24): warning C4067: unexpected tokens following preprocessor directive - expected a newline
6>..\platform\graphics\cairo\DrawErrorUnderline.h(24): warning C4067: unexpected tokens following preprocessor directive - expected a newline
6>InspectorIndexedDBAgent.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>InspectorFileSystemAgent.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>StorageInfo.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBTransaction.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBRequest.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBObjectStoreBackendImpl.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBObjectStore.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBKeyRange.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBKey.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBIndexBackendImpl.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBIndex.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBFactoryBackendInterface.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBFactoryBackendImpl.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBFactory.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBDatabaseBackendImpl.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBDatabase.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBCursorBackendImpl.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBCursor.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>IDBAny.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>PluginDebug.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSFileReaderCustom.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSEntrySyncCustom.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSEntryCustom.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSDirectoryEntrySyncCustom.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSDirectoryEntryCustom.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>ProgressShadowElement.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MediaControls.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MediaControlRootElement.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MediaControlElements.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>WeekInputType.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>TimeInputType.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>OperationNotAllowedException.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MonthInputType.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MicroDataItemValue.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MediaFragmentURIParser.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MediaDocument.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MediaController.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>LocalFileSystem.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>HTMLPropertiesCollection.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>HTMLParserErrorCodes.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileWriterSync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileWriterBase.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileWriter.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileThread.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileSystemCallbacks.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileStreamProxy.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileReaderSync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileReaderLoader.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileReader.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileException.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileEntrySync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileEntry.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>EntrySync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>EntryArraySync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>EntryArray.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>Entry.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DOMURL.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DOMFileSystemSync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DOMFileSystemBase.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DOMFileSystem.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DOMFilePath.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DirectoryReaderSync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DirectoryReader.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DirectoryEntrySync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DirectoryEntry.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DateTimeLocalInputType.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DateTimeInputType.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DateInputType.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>ColorInputType.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>NotificationCenter.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>Notification.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>ScriptedAnimationController.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>StyleCachedShader.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>RenderLayerBacking.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>RenderFullScreen.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>WebKitCSSShaderValue.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>WebKitCSSFilterValue.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>SpeechInputClientMock.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>LoaderRunLoopCF.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>BlobResourceHandle.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>BlobRegistryImpl.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MediaPlayerPrivateAVFoundationCF.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MediaPlayerPrivateAVFoundation.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>WKCACFViewLayerTreeHost.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>PlatformCALayerWinInternal.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>PlatformCALayerWin.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>PlatformCAAnimationWin.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>LegacyCACFLayerTreeHost.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>TransformationMatrixCA.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>GraphicsLayerCA.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FilterOperations.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FilterOperation.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FELightingNEON.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FEGaussianBlurNEON.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FECustomFilter.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FECompositeArithmeticNEON.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>CustomFilterShader.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>CustomFilterProgram.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>CustomFilterOperation.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>CustomFilterMesh.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FullScreenController.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MediaPlayer.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>GraphicsLayer.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>WebCorePrefix.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>ScrollbarThemeSafari.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>GDIObjectCounter.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>ScrollAnimatorWin.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>PlatformEvent.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>FileStream.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>CalculationValue.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>AsyncFileSystem.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>CachedShader.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MHTMLParser.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>MHTMLArchive.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>SpeechInput.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>PerformanceTiming.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>PerformanceNavigation.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>Performance.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>PageVisibilityState.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSStorageInfoUsageCallback.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSStorageInfoQuotaCallback.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSStorageInfoErrorCallback.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSStorageInfo.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSOperationNotAllowedException.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSFileWriterCallback.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSFileReaderSync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSFileException.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSFileEntrySync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSFileEntry.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSFileCallback.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSEntrySync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSEntryArraySync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSDOMFileSystemSync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSDirectoryReaderSync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>JSDirectoryEntrySync.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
6>DerivedSources.obj : warning LNK4006: "protected: void __thiscall WebCore::JSWebKitCSSRegionRule::finishCreation(class JSC::JSGlobalData &)" (?finishCreation@JSWebKitCSSRegionRule@WebCore@@IAEXAAVJSGlobalData@JSC@@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "private: __thiscall WebCore::JSWebKitCSSRegionRuleConstructor::JSWebKitCSSRegionRuleConstructor(class JSC::Structure *,class WebCore::JSDOMGlobalObject *)" (??0JSWebKitCSSRegionRuleConstructor@WebCore@@AAE@PAVStructure@JSC@@PAVJSDOMGlobalObject@1@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static bool __cdecl WebCore::JSWebKitCSSRegionRuleConstructor::getOwnPropertyDescriptor(class JSC::JSObject *,class JSC::ExecState *,class JSC::Identifier const &,class JSC::PropertyDescriptor &)" (?getOwnPropertyDescriptor@JSWebKitCSSRegionRuleConstructor@WebCore@@SA_NPAVJSObject@JSC@@PAVExecState@4@ABVIdentifier@4@AAVPropertyDescriptor@4@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "protected: __thiscall WebCore::JSWebKitCSSRegionRule::JSWebKitCSSRegionRule(class JSC::Structure *,class WebCore::JSDOMGlobalObject *,class WTF::PassRefPtr)" (??0JSWebKitCSSRegionRule@WebCore@@IAE@PAVStructure@JSC@@PAVJSDOMGlobalObject@1@V?$PassRefPtr@VWebKitCSSRegionRule@WebCore@@@WTF@@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static bool __cdecl WebCore::JSWebKitCSSRegionRuleConstructor::getOwnPropertySlot(class JSC::JSCell *,class JSC::ExecState *,class JSC::Identifier const &,class JSC::PropertySlot &)" (?getOwnPropertySlot@JSWebKitCSSRegionRuleConstructor@WebCore@@SA_NPAVJSCell@JSC@@PAVExecState@4@ABVIdentifier@4@AAVPropertySlot@4@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static bool __cdecl WebCore::JSWebKitCSSRegionRule::getOwnPropertyDescriptor(class JSC::JSObject *,class JSC::ExecState *,class JSC::Identifier const &,class JSC::PropertyDescriptor &)" (?getOwnPropertyDescriptor@JSWebKitCSSRegionRule@WebCore@@SA_NPAVJSObject@JSC@@PAVExecState@4@ABVIdentifier@4@AAVPropertyDescriptor@4@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static bool __cdecl WebCore::JSWebKitCSSRegionRule::getOwnPropertySlot(class JSC::JSCell *,class JSC::ExecState *,class JSC::Identifier const &,class JSC::PropertySlot &)" (?getOwnPropertySlot@JSWebKitCSSRegionRule@WebCore@@SA_NPAVJSCell@JSC@@PAVExecState@4@ABVIdentifier@4@AAVPropertySlot@4@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static class JSC::JSObject * __cdecl WebCore::JSWebKitCSSRegionRule::createPrototype(class JSC::ExecState *,class JSC::JSGlobalObject *)" (?createPrototype@JSWebKitCSSRegionRule@WebCore@@SAPAVJSObject@JSC@@PAVExecState@4@PAVJSGlobalObject@4@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static class JSC::JSObject * __cdecl WebCore::JSWebKitCSSRegionRulePrototype::self(class JSC::ExecState *,class JSC::JSGlobalObject *)" (?self@JSWebKitCSSRegionRulePrototype@WebCore@@SAPAVJSObject@JSC@@PAVExecState@4@PAVJSGlobalObject@4@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "private: void __thiscall WebCore::JSWebKitCSSRegionRuleConstructor::finishCreation(class JSC::ExecState *,class WebCore::JSDOMGlobalObject *)" (?finishCreation@JSWebKitCSSRegionRuleConstructor@WebCore@@AAEXPAVExecState@JSC@@PAVJSDOMGlobalObject@2@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static class JSC::JSValue __cdecl WebCore::JSWebKitCSSRegionRule::getConstructor(class JSC::ExecState *,class JSC::JSGlobalObject *)" (?getConstructor@JSWebKitCSSRegionRule@WebCore@@SA?AVJSValue@JSC@@PAVExecState@4@PAVJSGlobalObject@4@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "class JSC::JSValue __cdecl WebCore::jsWebKitCSSRegionRuleCssRules(class JSC::ExecState *,class JSC::JSValue,class JSC::Identifier const &)" (?jsWebKitCSSRegionRuleCssRules@WebCore@@YA?AVJSValue@JSC@@PAVExecState@3@V23@ABVIdentifier@3@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "class JSC::JSValue __cdecl WebCore::jsWebKitCSSRegionRuleConstructor(class JSC::ExecState *,class JSC::JSValue,class JSC::Identifier const &)" (?jsWebKitCSSRegionRuleConstructor@WebCore@@YA?AVJSValue@JSC@@PAVExecState@3@V23@ABVIdentifier@3@@Z) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static struct JSC::ClassInfo const WebCore::JSWebKitCSSRegionRuleConstructor::s_info" (?s_info@JSWebKitCSSRegionRuleConstructor@WebCore@@2UClassInfo@JSC@@B) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static struct JSC::ClassInfo const WebCore::JSWebKitCSSRegionRulePrototype::s_info" (?s_info@JSWebKitCSSRegionRulePrototype@WebCore@@2UClassInfo@JSC@@B) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
6>DerivedSources.obj : warning LNK4006: "public: static struct JSC::ClassInfo const WebCore::JSWebKitCSSRegionRule::s_info" (?s_info@JSWebKitCSSRegionRule@WebCore@@2UClassInfo@JSC@@B) already defined in JSWebKitCSSRegionRule.obj; second definition ignored
7>------ Build started: Project: Interfaces, Configuration: Release_Cairo_CFLite Win32 ------
8>------ Build started: Project: WebKitGUID, Configuration: Release_Cairo_CFLite Win32 ------
9>------ Build started: Project: WebKitLib, Configuration: Release_Cairo_CFLite Win32 ------
9>WebDesktopNotificationsDelegate.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
9>FullscreenVideoController.obj : warning LNK4221: This object file does not define any previously undefined public symbols, so it will not be used by any link operation that consumes this library
10>------ Build started: Project: WebKit2Generated, Configuration: Release_Cairo_CFLite Win32 ------
11>------ Build started: Project: WebKit, Configuration: Release_Cairo_CFLite Win32 ------
12>------ Build started: Project: WebKit2WebProcess, Configuration: Release_Cairo_CFLite Win32 ------
13>------ Build started: Project: testapi, Configuration: Release_Cairo_CFLite Win32 ------
14>------ Build started: Project: jsc, Configuration: Release_Cairo_CFLite Win32 ------
15>------ Build started: Project: testRegExp, Configuration: Release_Cairo_CFLite Win32 ------
16>------ Build started: Project: WinLauncher, Configuration: Release_Cairo_CFLite Win32 ------
17>------ Build started: Project: WinLauncherLauncher, Configuration: Release_Cairo_CFLite Win32 ------
17>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(990,5): warning MSB8012: TargetPath(C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\WinLauncherLauncher.exe) does not match the Linker's OutputFile property value (C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\WinLauncher.exe). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
17>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(992,5): warning MSB8012: TargetName(WinLauncherLauncher) does not match the Linker's OutputFile property value (WinLauncher). This may cause your project
to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
18>------ Build started: Project: TestNetscapePlugin, Configuration: Release_Cairo_CFLite Win32 ------
18>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(990,5): warning MSB8012: TargetPath(C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\TestNetscapePlugin.dll) does not match the Linker's OutputFile property value (C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\TestNetscapePlugin\npTestNetscapePlugin.dll). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
18>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(992,5): warning MSB8012: TargetName(TestNetscapePlugin) does not match the Linker's OutputFile property value (npTestNetscapePlugin). This may cause your
project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
19>------ Build started: Project: ImageDiff, Configuration: Release_Cairo_CFLite Win32 ------
19>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(990,5): warning MSB8012: TargetPath(C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\ImageDiff.dll) does not match the Linker's OutputFile property value (C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\ImageDiff.exe). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
19>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(991,5): warning MSB8012: TargetExt(.dll) does not match the Linker's OutputFile property value (.exe). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
20>------ Build started: Project: ImageDiffLauncher, Configuration: Release_Cairo_CFLite Win32 ------
20>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(990,5): warning MSB8012: TargetPath(C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\ImageDiffLauncher.exe) does not match the Linker's OutputFile property value (C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\ImageDiff.exe). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
20>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(992,5): warning MSB8012: TargetName(ImageDiffLauncher) does not match the Linker's OutputFile property value (ImageDiff). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
21>------ Build started: Project: WebCoreTestSupport, Configuration: Release_Cairo_CFLite Win32 ------
22>------ Build started: Project: DumpRenderTree, Configuration: Release_Cairo_CFLite Win32 ------
23>------ Build started: Project: DumpRenderTreeLauncher, Configuration: Release_Cairo_CFLite Win32 ------
23>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(990,5): warning MSB8012: TargetPath(C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\DumpRenderTreeLauncher.exe) does not match the
Linker's OutputFile property value (C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\DumpRenderTree.exe). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
23>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(992,5): warning MSB8012: TargetName(DumpRenderTreeLauncher) does not match the Linker's OutputFile property value (DumpRenderTree). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
24>------ Build started: Project: WebKitLauncherWin, Configuration: Release_Cairo_CFLite Win32 ------
24>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(990,5): warning MSB8012: TargetPath(C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\WebKitLauncherWin.exe) does not match the Linker's OutputFile property value (C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\WebKit.exe). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
24>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(992,5): warning MSB8012: TargetName(WebKitLauncherWin) does not match the Linker's OutputFile property value (WebKit). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
25>------ Build started: Project: record-memory-win, Configuration: Release_Cairo_CFLite Win32 ------
26>------ Build started: Project: InjectedBundleGenerated, Configuration: Release_Cairo_CFLite Win32 ------
27>------ Build started: Project: InjectedBundle, Configuration: Release_Cairo_CFLite Win32 ------
28>------ Build started: Project: WebKitTestRunner, Configuration: Release_Cairo_CFLite Win32 ------
29>------ Build started: Project: WebKitTestRunnerLauncher, Configuration: Release_Cairo_CFLite Win32 ------
29>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(990,5): warning MSB8012: TargetPath(C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\WebKitTestRunnerLauncher.exe) does not match the Linker's OutputFile property value (C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\WebKitTestRunner.exe). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
29>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(992,5): warning MSB8012: TargetName(WebKitTestRunnerLauncher) does not match the Linker's OutputFile property value (WebKitTestRunner). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
30>------ Build started: Project: gtest-md, Configuration: Release_Cairo_CFLite Win32 ------
30>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(1151,5): warning MSB8012: TargetPath(C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\lib\gtest-md.lib) does not match the Library's OutputFile property value (C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\lib\gtest.lib). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Lib.OutputFile).
30>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(1153,5): warning MSB8012: TargetName(gtest-md) does not match the Library's OutputFile property value (gtest). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Lib.OutputFile).
31>------ Build started: Project: TestWebKitAPIGenerated, Configuration: Release_Cairo_CFLite Win32 ------
32>------ Build started: Project: TestWebKitAPI, Configuration: Release_Cairo_CFLite Win32 ------
33>------ Build started: Project: TestWebKitAPIInjectedBundle, Configuration: Release_Cairo_CFLite Win32 ------
34>------ Build started: Project: MiniBrowser, Configuration: Release_Cairo_CFLite Win32 ------
35>------ Build started: Project: MiniBrowserLauncher, Configuration: Release_Cairo_CFLite Win32 ------
35>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(990,5): warning MSB8012: TargetPath(C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\MiniBrowserLauncher.exe) does not match the Linker's OutputFile property value (C:\Cygwin\home\Ash\WebKit-r106194\WebKitBuild\Release_Cairo_CFLite\bin\MiniBrowser.exe). This may cause your project to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
35>C:\Program Files (x86)\MSBuild\Microsoft.Cpp\v4.0\Microsoft.CppBuild.targets(992,5): warning MSB8012: TargetName(MiniBrowserLauncher) does not match the Linker's OutputFile property value (MiniBrowser). This may cause your project
to build incorrectly. To correct this, please make sure that $(OutDir), $(TargetName) and $(TargetExt) property values match the value specified in %(Link.OutputFile).
========== Build: 34 succeeded, 0 failed, 0 up-to-date, 1 skipped ==========
===========================================================
WebKit is now built (07m:41s).
To run Safari with this newly-built code, use the
"../../../Cygwin/home/Ash/WebKit-r106194/Tools/Scripts/run-safari" script.
===========================================================
Astute readers might notice that my previous build took 49m:07s while this one is only 07m:41s. This is thanks to my new 6-core i7 3930K @ 4.6 Ghz, 32 GB 1600 @ CL9 on 256 GB Samsung 830 SSD. The previous build was on Core 2 Quad 9300 @ 2.7 Ghz, 4 GB 800 @ CL5 on 150 GB WD VelociRaptor @ 10,000 (with RamDisk). That’s an almost 6.5x difference.
The dos2unix
entries are auto-conversion of Bash files that often don’t have correct line-ending, which used to break the build on some Cygwin Bash instances. This takes a couple of extra seconds but ensures that no line-ending discrepancy exists.
Latest Revision
Now it’s time to try the latest revision. It’s interesting to see what difference do 20,000 revisions and almost 1000 new files make. After extracting the archive, I simply copied the contents of my patch (2 files) overwriting pdevenv
. I made sure that no instance of MSBuild.exe was running.
Microsoft (R) Microsoft Visual Studio 2012 Version 11.0.50727.1.
Copyright (C) Microsoft Corp. All rights reserved.
1>------ Build started: Project: WTFGenerated, Configuration: Release_Cairo_CFLite Win32 ------
2>------ Build started: Project: WTF (WTF\WTF), Configuration: Release_Cairo_CFLite Win32 ------
3>------ Build started: Project: JavaScriptCoreGenerated, Configuration: Release_Cairo_CFLite Win32 ------
4>------ Build started: Project: JavaScriptCore, Configuration: Release_Cairo_CFLite Win32 ------
5>------ Build started: Project: WebCoreGenerated, Configuration: Release_Cairo_CFLite Win32 ------
5>Z:\WebKit-r127371\Source\WebCore\WebCore.vcproj\WebCoreGeneratedCairo.props(4,5): warning MSB4011: "Z:\WebKit-r127371\WebKitLibraries\win\tools\vsprops\common.props" cannot be imported again. It was already imported at "Z:\WebKit-r127371\Source\WebCore\WebCore.vcproj\WebCoreGeneratedCommon.props (4,5)". This is most likely a build authoring error. This subsequent import will be ignored. [Z:\WebKit-r127371\Source\WebCore\WebCore.vcproj\WebCoreGenerated.vcxproj]
6>------ Skipped Build: Project: QTMovieWin, Configuration: Release_Cairo_CFLite Win32 ------
6>Project not selected to build for this solution configuration
7>------ Build started: Project: WebCore, Configuration: Release_Cairo_CFLite Win32 ------
7>c1xx : fatal error C1083: Cannot open source file: '..\storage\StorageInfo.cpp': No such file or directory
7>..\platform\graphics\cairo\DrawErrorUnderline.h(24): warning C4067: unexpected tokens following preprocessor directive - expected a newline
I might have been a bit overoptimistic, but I really was hoping it’d build. There seems to be missing source files. First assumption is that I got unlucky with the revision. After building over half a dozen revisions I was convinced it wasn’t a fluke. I checked the repository and sure enough the file is not there. Digging further I found a rather old version of the file. Circa April 2011. Further investigations showed that the file in question is a port-specific implementation. Chromium has its own implementation. But this version is the default. My best explanation is that the last major port on Windows was Safari, and they stopped supporting Windows. So that leaves Chromium and they already have a specialized implementation in their port-specific folder/project. Whatever the case, I restored the last implementation I could find, which doesn’t do anything really.
Placing the missing files gives us a successful build.
Visual Studio 2012
I thought it’d be fun to try and see how VS2012 would fare. I was encouraged after reading that the latest version of the popular IDE finally creates almost backwards compatible solution and project files. I say almost because, well, it’s not perfect. Another minor push came from the fact that Windows 8 SDK is included and DirectX SDK as well. That meant less setup hassle.
First Impressions: A Mini Review
Visual Studio certainly warrants a full review in its own right, but a quick overview will do here. First thing to notice was that it loaded very swiftly. Projects loaded faster as well. Mind you, I also thought it must be my new hardware at work. But VS2010 doesn’t load nearly as fast. Next was a small disappointment as I stared at the drab looking GUI. It was ’97 all over again. Everything is grey. Icons are grey too. Are we that desensitized by colors that we want to do away with them? I found finding commands in the menus difficult as my eyes were struggling to find anything familiar at sight without reading each entry.
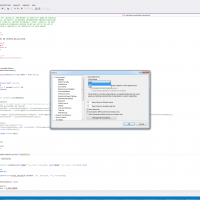
First thing I did was to go to the Options dialog. To my sweet surprise, there is a dark theme! I almost exclusively use dark themes in my editors for lesser eye strain. The high contrast of black-on-white isn’t very relaxing to the eye nerves, especially after long hours. Dark themes help by lowering the contrast. Native support of dark themes is very important because some plugins assumed that the background was always light-colored. So their default choices of text color has been dark colors. This can be fixed in most cases, but some text colors aren’t configurable. I immediately switched to dark theme expecting a restart, to my surprise it instantly redrew the whole application without a single blemish. VS2010 couldn’t even change the background color of its Solution window (it wasn’t ported from Win32 to WPF).
Next I noticed that (finally) variables are highlighted when the cursor is placed on them. This has been in Notepad++ for ages. VS2012 chose to support highlighting variables within the current context. So that also means only variables are highlighted. One can’t highlight all new
keywords, for example, to see all allocations at a glance. Still, it’s an improvement.
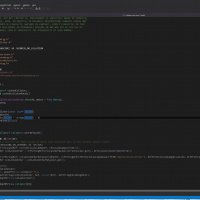
Things aren’t perfect, of course. There is always a catch. After running VS2012 on a project in my RamDisk I noticed that Intellisense stopped working. With it, highlighting went away. I tried everything and the only change is RamDisk. When the project is loaded from RamDisk, Intellisense files aren’t created or created with a minimal size. Visual Studio complains upon closing (and of course upon invoking any Intellisense comand) that it couldn’t load Intellisense files. Obviously a bug.
Another sweet feature that I noticed right off the bat is the “quick preview” (as I call it) feature. Basically, selecting any file shows the file in a single tab to the far right which is recycled. Double click to open a permanent tab or click on the “Keep Open” button on the temporary one. This works best from the Find window where one can quickly go through the found lines by the cursor and preview the lines in context without cluttering the tabs.
Btw, notice the solution loaded is VS2010 converted. It didn’t need any modifications to load (it loaded silently). I don’t know if incompatible changes are made when project settings are changed.
Building with VS2012
It was really going great for the longest time, until it didn’t. MIDL found an indefensible statement:
Microsoft (R) Microsoft Visual Studio 2012 Version 11.0.50727.1.
Copyright (C) Microsoft Corp. All rights reserved.
1>------ Build started: Project: WTFGenerated, Configuration: Release_Cairo_CFLite Win32 ------
2>------ Build started: Project: WTF (WTF\WTF), Configuration: Release_Cairo_CFLite Win32 ------
3>------ Build started: Project: JavaScriptCoreGenerated, Configuration: Release_Cairo_CFLite Win32 ------
4>------ Build started: Project: JavaScriptCore, Configuration: Release_Cairo_CFLite Win32 ------
5>------ Build started: Project: WebCoreGenerated, Configuration: Release_Cairo_CFLite Win32 ------
5>Z:\WebKit-r127371\Source\WebCore\WebCore.vcproj\WebCoreGeneratedCairo.props(4,5): warning MSB4011: "Z:\WebKit-r127371\WebKitLibraries\win\tools\vsprops\common.props" cannot be imported again. It was already imported at "Z:\WebKit-r127371\Source\WebCore\WebCore.vcproj\WebCoreGeneratedCommon.props (4,5)". This is most likely a build authoring error. This subsequent import will be ignored. [Z:\WebKit-r127371\Source\WebCore\WebCore.vcproj\WebCoreGenerated.vcxproj]
6>------ Skipped Build: Project: QTMovieWin, Configuration: Release_Cairo_CFLite Win32 ------
6>Project not selected to build for this solution configuration
7>------ Build started: Project: WebCore, Configuration: Release_Cairo_CFLite Win32 ------
8>------ Build started: Project: Interfaces, Configuration: Release_Cairo_CFLite Win32 ------
8>MIDL : error MIDL2337: unsatisfied forward declaration : WebFontDescription
9>------ Build started: Project: WebKitGUID, Configuration: Release_Cairo_CFLite Win32 ------
The MIDL version of the SDK that ships with VS2012 doesn’t like forward declaring without defining. I replaced the offending typedef with a simpler forward declaration. To automate the modification, I updated pdevenv
with the appropriate Perl scripts.
# Patch DOMPrivate.idl.
print $fh "\@echo Patching DOMPrivate.idl...\r\n";
my $idl_filename = $webkitRootPath . "\\Source\\WebKit\\win\\Interfaces\\DOMPrivate.idl";
chomp($idl_filename = `cygpath -w -a '$idl_filename'`);
my $replace_string = "s/typedef struct WebFontDescription WebFontDescription/struct WebFontDescription/g";
print $fh "perl -p -i -e \"" . $replace_string . "\" \"" . $idl_filename . "\"\r\n";
$replace_string = "s/\(WebFontDescription\\* webFontDescription/\(struct WebFontDescription\\* webFontDescription/g";
print $fh "perl -p -i -e \"" . $replace_string . "\" \"" . $idl_filename . "\"\r\n";
Next set of errors came from the Linker. Missing functions. A quick look reminded me of VS2010 project conversion errors. In previous version of Visual Studio the project files had an entry per file and nested within that entry were the configuration-specific nodes. In VS2010 and VS2012 this has changed to a tool-specific node that has a file as its attribute. Because ignored files in the original VS2005 project had their tool set to custom, upon conversion VS2010 and VS2012 have decided that the relevant files are custom files, although they are C++ sources. To add insult to injury, VS2012 has changed the custom tool tag-name from CustomBuildStep
to CustomBuild
.
$replace_string = "undef \$/; s/\\<CustomBuild(.*?)\\.cpp(.*?)CustomBuild\\>/\\<ClCompile\$1\\.cpp\$2ClCompile\\>/msgi";
print $fh "perl -0pi -e \"" . $replace_string . "\" \"" . $webcore_filename . "\"\r\n";
Next the complaints came from Tuple. This is most odd because one would expect newer versions of the compiler to have better support of the standard, not worse.
31>Z:\WebKit-r127371\Source\ThirdParty\gtest\include\gtest/internal/gtest-param-util-generated.h(4015): error C2977: 'std::tuple' : too many template arguments (..\src\gtest-typed-test.cc)
31>Z:\WebKit-r127371\Source\ThirdParty\gtest\include\gtest/internal/gtest-param-util-generated.h(4015): error C3203: 'tuple' : unspecialized class template can't be used as a template argument for template parameter 'T', expected a real type (..\src\
test-typed-test.cc)
31>Z:\WebKit-r127371\Source\ThirdParty\gtest\include\gtest/internal/gtest-param-util-generated.h(4015): error C2955: 'std::tuple' : use of class template requires template argument list (..\src\gtest-typed-test.cc)
31>Z:\WebKit-r127371\Source\ThirdParty\gtest\include\gtest/internal/gtest-param-util-generated.h(4015): error C2955: 'testing::internal::ParamGeneratorInterface' : use of class template requires template argument list (..\src\gtest-typed-test.cc)
31>Z:\WebKit-r127371\Source\ThirdParty\gtest\include\gtest/internal/gtest-param-util-generated.h(4017): error C2977: 'std::tuple' : too many template arguments (..\src\gtest-typed-test.cc)
31>Z:\WebKit-r127371\Source\ThirdParty\gtest\include\gtest/internal/gtest-param-util-generated.h(4028): error C3203: 'tuple' : unspecialized class template can't be used as a template argument for template parameter 'T', expected a real type (..\src\
test-typed-test.cc)
31>Z:\WebKit-r127371\Source\ThirdParty\gtest\include\gtest/internal/gtest-param-util-generated.h(4028): error C2955: 'std::tuple' : use of class template requires template argument list (..\src\gtest-typed-test.cc)
Anyway, noticing that this was indeed test project, and the errors (too many to list here) coming from gtest, I thought it fair to call it a day and let Google worry about bringing gtest up to speed with VS2012. As far as I was concerned, I build WebKit on VS2012, sans the tests, of course.
Full Script
#!/usr/bin/perl -w
use strict;
use warnings;
use File::Temp qw/tempfile/;
use File::Copy;
use File::Find;
use FindBin;
use lib $FindBin::Bin;
use webkitdirs;
# Visual Studio is executed using a temp Windows Batch file.
my ($fh, $path) = tempfile(UNLINK => 0, SUFFIX => '.cmd') or die;
print $fh "\@echo off\r\n"; # Comment-out to see/debug executed commands.
chomp(my $vcBin = `cygpath -w "$FindBin::Bin/../vcbin"`);
chomp(my $scriptsPath = `cygpath -w "$FindBin::Bin"`);
my $webkitRootPath = $scriptsPath . "\\..\\..";
my $originalWorkingDirectory = getcwd();
print "Current working directory: " . $originalWorkingDirectory . "\n";
# Cygwin Bash expects unix files (LF only).
chomp(my $root = `cygpath "$webkitRootPath"`);
system("dos2unix " . $root . "autogen.sh");
find( sub { if (/\.sh$/) { my $fname = `cygpath "$File::Find::name$/"`; `dos2unix $fname` } }, $webkitRootPath . "\\Source");
find( sub { if (/\.sh$/) { my $fname = `cygpath "$File::Find::name$/"`; `dos2unix $fname` } }, $webkitRootPath . "\\Tools");
find( sub { if (/\.sh$/) { my $fname = `cygpath "$File::Find::name$/"`; `dos2unix $fname` } }, $webkitRootPath . "\\WebKitLibraries");
my $jsdef_filename = $webkitRootPath . "\\Source\\JavaScriptCore\\JavaScriptCore.vcproj\\JavaScriptCore\\JavaScriptCore.def";
chomp($jsdef_filename = `cygpath -w -a '$jsdef_filename'`);
my $command = join(" ", @ARGV);
my $vsToolsVar;
sub Fixup
{
my ($versionName, $vsToolsVarName, $solution) = @_;
$solution =~ s/\\/\//g; # Avoid having backslash because they are also escapes.
chomp($solution = `cygpath -w -a $solution`);
my $origSolution = $solution;
$solution =~ s/\.sln/-$versionName\.sln/g;
print $fh "if not exist " . $webkitRootPath . "\\WebKitLibraries ( \@echo This is not a WebKit directory! Stopping.\n goto exit )\r\n";
print $fh "if not exist " . $solution . " (\r\n";
print $fh "copy " . $origSolution . " " . $solution . "\r\n";
print $fh ")\r\n"; # If no converted solution.
print $fh "\@echo Upgrading solution file @ " . $solution . ". Delete this file to force-upgrade all projects.\r\n";
print $fh "\@echo Build will commence when done. Please be patient.\r\n";
print $fh "call \"\%" . $vsToolsVarName . "\%\\vsvars32.bat\"\r\n";
print $fh "IF EXIST \"\%VSINSTALLDIR\%\\Common7\\IDE\\devenv.com\" (devenv.com /useenv /upgrade " . join(" ", $solution) . ") ELSE ";
print $fh "VCExpress.exe /useenv /upgrade " . join(" ", $solution) . "\r\n";
# Correct the solution file name.
$command = '';
foreach my $arg (@ARGV) {
$arg =~ s/\.sln/-$versionName\.sln/g;
$command = $command . ' ' . $arg;
}
# Patch DOMPrivate.idl.
print $fh "\@echo Patching DOMPrivate.idl...\r\n";
my $idl_filename = $webkitRootPath . "\\Source\\WebKit\\win\\Interfaces\\DOMPrivate.idl";
chomp($idl_filename = `cygpath -w -a '$idl_filename'`);
my $replace_string = "s/typedef struct WebFontDescription WebFontDescription/struct WebFontDescription/g";
print $fh "perl -p -i -e \"" . $replace_string . "\" \"" . $idl_filename . "\"\r\n";
$replace_string = "s/\(WebFontDescription\\* webFontDescription/\(struct WebFontDescription\\* webFontDescription/g";
print $fh "perl -p -i -e \"" . $replace_string . "\" \"" . $idl_filename . "\"\r\n";
# Patch common.props.
print $fh "\@echo Patching common.props...\r\n";
my $props_filename = $webkitRootPath . "\\WebKitLibraries\\win\\tools\\vsprops\\common.props";
chomp($props_filename = `cygpath -w -a '$props_filename'`);
print $fh "\@echo Disabling warning as error.\r\n";
$replace_string = "s/\\true\\<\\/TreatWarningAsError\\>/\\false\\<\\/TreatWarningAsError\\>/g";
print $fh "perl -p -i -e \"" . $replace_string . "\" \"" . $props_filename . "\"\r\n";
print $fh "\@echo Disabling warning C4396.\r\n";
$replace_string = "s/\\<\\DisableSpecificWarnings\\>4396;/\\<\\DisableSpecificWarnings\\>/g"; # Delete disabled warning 4396.
print $fh "perl -p -i -e \"" . $replace_string . "\" \"" . $props_filename . "\"\r\n";
$replace_string = "s/\\<\\DisableSpecificWarnings\\>/\\<\\DisableSpecificWarnings\\>4396;/g";
print $fh "perl -p -i -e \"" . $replace_string . "\" \"" . $props_filename . "\"\r\n"; # Disable warning 4396.
print $fh "\@echo Enabling multiprocess compile.\r\n";
$replace_string = "s/\\(.*?)\\<\\/MultiProcessorCompilation\\>//g"; # Delete MP compilation.
print $fh "perl -p -i -e \"" . $replace_string . "\" \"" . $props_filename . "\"\r\n";
$replace_string = "s/\\<\\/DisableSpecificWarnings\\>/\\<\\/DisableSpecificWarnings\\>\\n \\true\\<\\/MultiProcessorCompilation\\>/g";
print $fh "perl -p -i -e \"" . $replace_string . "\" \"" . $props_filename . "\"\r\n"; # Enable MP compilation.
# Remove nullptr_t from JavaScriptCore.def
print $fh "\@echo Patching JavaScriptCore.def...\r\n";
print $fh "move \"" . $jsdef_filename . "\" \"" . $jsdef_filename . ".orig\" \r\n";
print $fh "findstr /V ?nullptr\@\@3Vnullptr_t\@std\@\@A \"" . $jsdef_filename . ".orig\" > \"" . $jsdef_filename . "\"\r\n";
my $webcore_filename = $webkitRootPath . "\\Source\\WebCore\\WebCore.vcproj\\WebCore.vcxproj";
chomp($webcore_filename = `cygpath -w -a '$webcore_filename'`);
print $fh "\@echo Correcting WebCore.vcxproj...\r\n";
$replace_string = "undef \$/; s/\\/\\/msgi";
print $fh "perl -0pi -e \"" . $replace_string . "\" \"" . $webcore_filename . "\"\r\n";
$replace_string = "undef \$/; s/\\/\\/msgi";
print $fh "perl -0pi -e \"" . $replace_string . "\" \"" . $webcore_filename . "\"\r\n";
}
if ($ENV{'VS110COMNTOOLS'}) {
$vcBin = ''; # We no longer need cl and midl wrappers.
$vsToolsVar = "VS110COMNTOOLS";
my $versionName = "vs2012";
print $fh "\@echo Using $versionName toolchain.\r\n";
print $fh "\@echo.\r\n";
# If there is no converted solution, upgrade.
my $solution = $ARGV[0];
if ($solution)
{
Fixup($versionName, $vsToolsVar, $solution);
}
} elsif ($ENV{'VS100COMNTOOLS'}) {
$vcBin = ''; # We no longer need cl and midl wrappers.
$vsToolsVar = "VS100COMNTOOLS";
my $versionName = "vs2010";
print $fh "\@echo Using $versionName toolchain.\r\n";
print $fh "\@echo.\r\n";
# If there is no converted solution, upgrade.
my $solution = $ARGV[0];
if ($solution)
{
Fixup($versionName, $vsToolsVar, $solution);
}
} elsif ($ENV{'VS90COMNTOOLS'}) {
$vsToolsVar = "VS90COMNTOOLS";
# Restore JavaScriptCore.def backup.
print $fh "if exist \"" . $jsdef_filename . ".orig\" (\r\n";
print $fh "\@echo Restoring JavaScriptCore.def...\r\n";
print $fh "move /Y \"" . $jsdef_filename . ".orig\" \"" . $jsdef_filename . "\" \r\n";
print $fh ")\r\n"; # If JavaScriptCore.def.orig.
} elsif ($ENV{'VS80COMNTOOLS'}) {
$vsToolsVar = "VS80COMNTOOLS";
# Restore JavaScriptCore.def backup.
print $fh "if exist \"" . $jsdef_filename . ".orig\" (\r\n";
print $fh "\@echo Restoring JavaScriptCore.def...\r\n";
print $fh "move /Y \"" . $jsdef_filename . ".orig\" \"" . $jsdef_filename . "\" \r\n";
print $fh ")\r\n"; # If JavaScriptCore.def.orig.
} else {
print "*************************************************************\n";
print "Cannot find Visual Studio tools dir.\n";
print "Please ensure that \$VS80COMNTOOLS, \$VS90COMNTOOLS or \$VS100COMNTOOLS\n";
print "is set to a valid location.\n";
print "*************************************************************\n";
die;
}
print $fh "call \"\%" . $vsToolsVar . "\%\\vsvars32.bat\"\r\n";
print $fh "set PATH=$vcBin;$scriptsPath;\%PATH\%\r\n";
print $fh "IF EXIST \"\%VSINSTALLDIR\%\\Common7\\IDE\\devenv.com\" (devenv.com /useenv " . join(" ", $command) . ") ELSE ";
print $fh "VCExpress.exe /useenv " . join(" ", $command) . "\r\n";
print $fh ":exit\n";
close $fh;
chmod 0755, $path;
chomp($path = `cygpath -w -s '$path'`);
# Fix up any env variables that differ only in case.
foreach my $key (sort keys(%ENV)) {
my $upper_key = uc($key);
my $dup = $ENV{$upper_key};
if ($dup && ($dup ne $ENV{$key}))
{
# Dups break CL.exe, so we need to remove either one. Since Cygwin seems to make Windows-inherited
# entries lower-case, and since CL plays better with Windows paths, we remove the upper-case one.
# There seems to be a bug in MSBuild.exe in that stays resident across builds, so environment
# variables changes aren't applied to future builds unless it's killed to force respawning.
print "Found duplicate env-var (rm $upper_key): \t$key = $ENV{$key}\t\t$upper_key = $dup\n";
delete $ENV{$upper_key};
}
}
my $batch_command = "cmd /c \"call $path\"";
print "Running $batch_command\n";
print "\@echo.\r\n";
system($batch_command);
unlink($path); # Comment-out to preserve the batch file for debugging.
What’s Next?
It’d be great if all the test projects also compiled. I’m sure in time this will get fixed. Another thing that I’d like to tackle is building the now-discontinued CoreGraphics on Windows. I expect this to be more of a challenge as the port rots. Still, it’s interesting. Finally, I’ll start maintaining a fork of WebKit where these fixes could be applied (yes, I tried, it’s really hard to get any unsupported code into the trunk).
Conclusion (and Patch)
Building WebKit is always challenging (and fun). Building the latest revision from scratch wasn’t trivial, but previous experience helped quite a bit. Moving to VS2012 wasn’t a breeze either, but it wasn’t nearly as hard as getting the first successful build with VS2010. Now, armed with this walk-through, one only needs to download the patch and extract it in the root of a recent revisions of a WebKit source tree for an easy and (hopefully) successful build.
Don’t shy from sharing your experience. Happy Hacking.